JavaScript Cheat Sheet: Essential Code Snippets
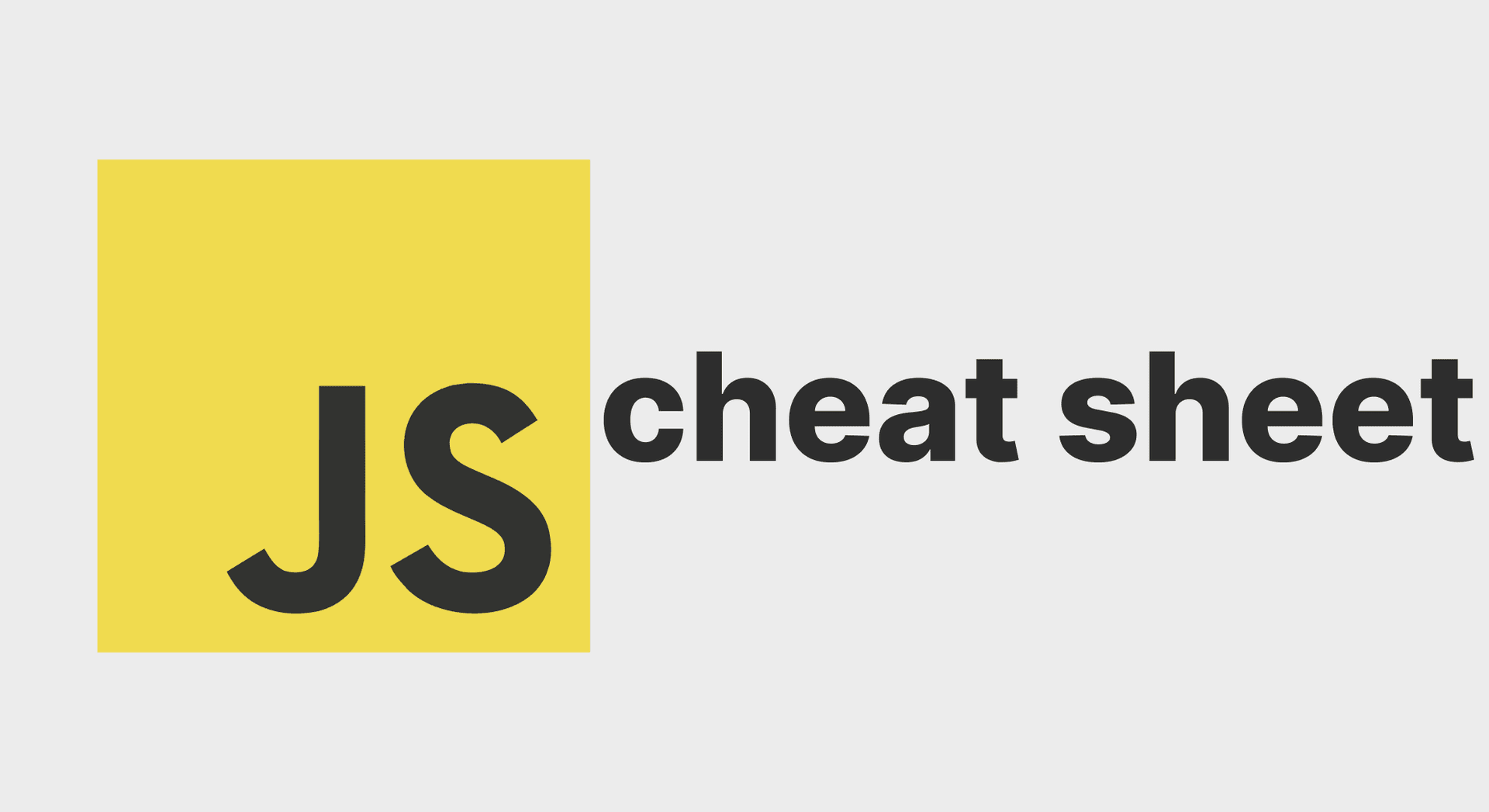
JavaScript Cheat Sheet: Essential Code Snippets
JavaScript is a versatile and widely used programming language that powers the web. This cheat sheet provides a compilation of essential code snippets for common functions, making it a handy reference for both beginners and experienced developers.
Working with Arrays
Iterate Over an Array
const numbers = [1, 2, 3, 4, 5];
for (const number of numbers) {
console.log(number);
}
Map an Array
const doubledNumbers = numbers.map(number => number * 2);
Filter an Array
const evenNumbers = numbers.filter(number => number % 2 === 0);
Reduce an Array
const sum = numbers.reduce((total, number) => total + number, 0);
Manipulating Strings
Concatenate Strings
const firstName = 'John';
const lastName = 'Doe';
const fullName = firstName + ' ' + lastName;
Convert to Uppercase or Lowercase
const text = 'Hello, World!';
const upperText = text.toUpperCase();
const lowerText = text.toLowerCase();
Check if String Contains a Substring
const sentence = 'JavaScript is amazing!';
const containsWord = sentence.includes('amazing');
Working with Objects
Create an Object
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30
};
Access Object Properties
const firstName = person.firstName;
const age = person['age'];
Iterate Over Object Properties
for (const key in person) {
console.log(key, person[key]);
}
Functions and Arrow Functions
Declare a Function
function greet(name) {
return 'Hello, ' + name + '!';
}
Arrow Function
const greet = name => `Hello, ${name}!`;
Default Parameters
function welcome(name = 'Guest') {
return 'Welcome, ' + name + '!';
}
Promises and Asynchronous Programming
Create a Promise
const fetchData = new Promise((resolve, reject) => {
// Fetch data asynchronously
if (data) {
resolve(data);
} else {
reject('Error fetching data');
}
});
Async/Await
async function fetchUser() {
try {
const response = await fetch('/api/user');
const user = await response.json();
return user;
} catch (error) {
console.error('Error fetching user:', error);
}
}
DOM Manipulation
Select an Element
const element = document.querySelector('.container');
Add Event Listener
element.addEventListener('click', event => {
console.log('Element clicked:', event.target);
});
Modify Element Content
element.textContent = 'New content';
Conclusion
This JavaScript cheat sheet covers a variety of essential code snippets for common programming tasks. Whether you're working with arrays, strings, objects, or dealing with asynchronous programming and DOM manipulation, these snippets will serve as valuable references as you build your JavaScript projects.