PyTorch Tensor Operations Cheat Sheet
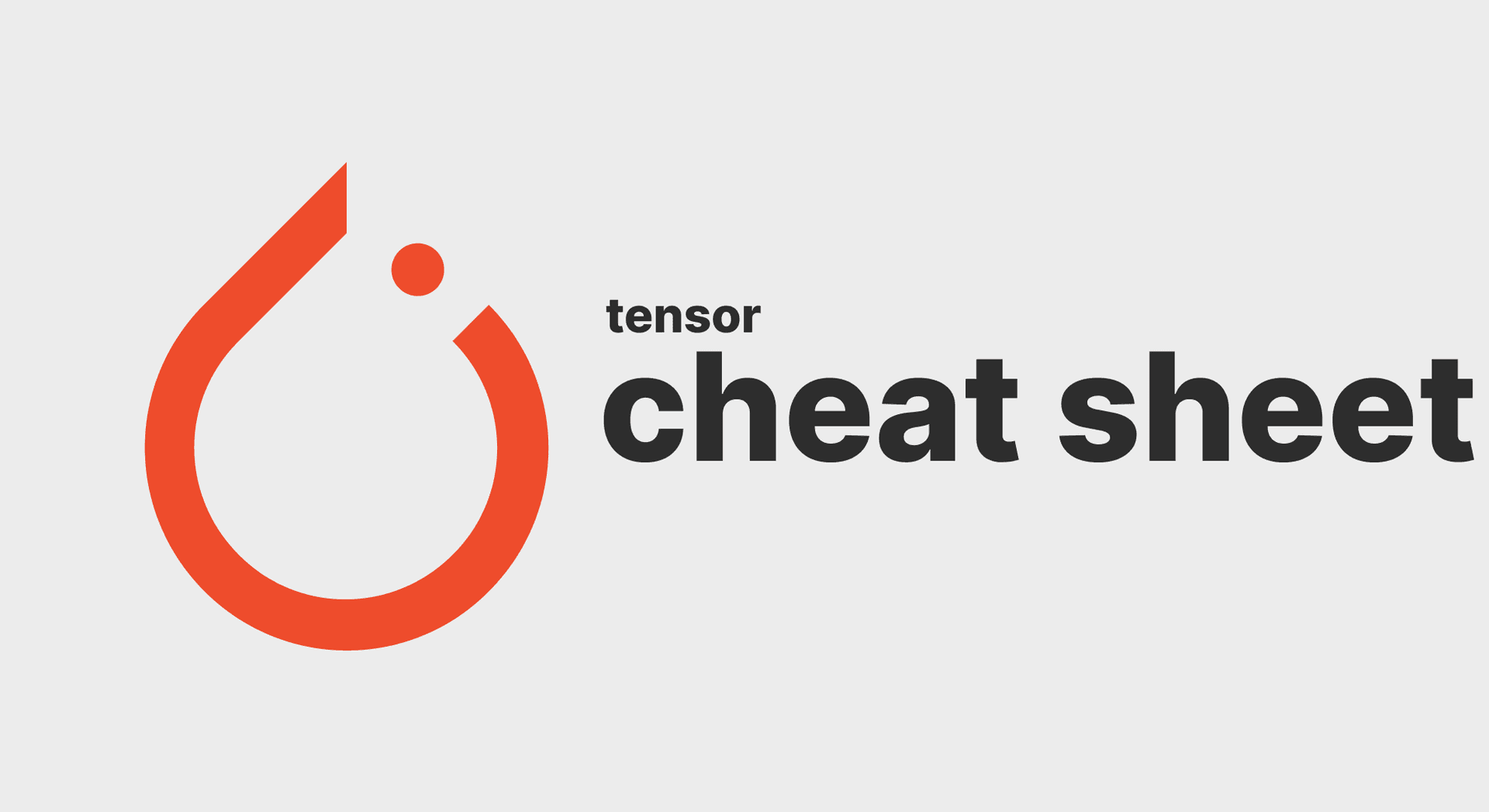
PyTorch Tensor Operations Cheat Sheet
Creating Tensors
-
Create a tensor:
tensor = torch.tensor([1, 2, 3])
-
Create a zero tensor:
zeros = torch.zeros(3, 3)
-
Create an identity matrix:
identity = torch.eye(3)
-
Create a tensor with random values:
rand_tensor = torch.rand(3, 3)
-
Create a tensor with specific range:
range_tensor = torch.arange(0, 10, 2)
Indexing and Slicing
-
Index a tensor:
element = tensor[0]
-
Slice a tensor:
slice = tensor[1:3]
-
Access rows/columns:
row = tensor[0, :] col = tensor[:, 0]
Shape Manipulation
-
Get tensor shape:
shape = tensor.shape
-
Reshape tensor:
reshaped = tensor.view(2, 2)
-
Flatten tensor:
flattened = tensor.flatten()
Arithmetic Operations
-
Addition:
result = tensor1 + tensor2
-
Subtraction:
result = tensor1 - tensor2
-
Multiplication:
result = tensor1 * tensor2
-
Division:
result = tensor1 / tensor2
Element-wise Operations
-
Element-wise square root:
sqrt = torch.sqrt(tensor)
-
Element-wise exponentiation:
exp = torch.exp(tensor)
-
Element-wise logarithm:
log = torch.log(tensor)
-
Element-wise absolute value:
abs = torch.abs(tensor)
Reduction Operations
-
Sum of all elements:
sum = tensor.sum()
-
Mean of all elements:
mean = tensor.mean()
-
Maximum element:
max_val = tensor.max()
-
Minimum element:
min_val = tensor.min()
Matrix Operations
-
Matrix multiplication:
matmul = torch.matmul(matrix1, matrix2)
-
Element-wise matrix multiplication:
element_wise = matrix1 * matrix2
-
Matrix transposition:
transposed = matrix.T
-
Matrix inverse:
inverse = torch.inverse(matrix)
Broadcasting
-
Expand tensor dimensions:
expanded = tensor.unsqueeze(0)
-
Broadcast tensors for element-wise operations:
result = tensor1 + tensor2
GPU Acceleration
-
Move tensor to GPU:
tensor.to('cuda')
-
Perform operations on GPU:
result = tensor1 + tensor2
Conversion
-
Convert to NumPy array:
numpy_array = tensor.numpy()
-
Convert to Python scalar:
scalar = tensor.item()
Concatenation and Stacking
-
Concatenate tensors along a dimension:
concatenated = torch.cat([tensor1, tensor2], dim=0)
-
Stack tensors along a new dimension:
stacked = torch.stack([tensor1, tensor2], dim=0)
Element-wise Comparison
-
Element-wise equality:
equal = torch.eq(tensor1, tensor2)
-
Element-wise greater than:
gt = torch.gt(tensor1, tensor2)
-
Element-wise less than or equal:
le = torch.le(tensor1, tensor2)
Element-wise Logic Operations
-
Element-wise logical AND:
logical_and = tensor1 & tensor2
-
Element-wise logical OR:
logical_or = tensor1 | tensor2
-
Element-wise logical NOT:
logical_not = ~tensor
Random Number Generation
-
Generate random tensor from uniform distribution:
uniform = torch.rand(3, 3)
-
Generate random tensor from normal distribution:
normal = torch.randn(3, 3)
Element-wise Round Operations
-
Round elements to nearest integer:
rounded = torch.round(tensor)
-
Floor elements to nearest integer:
floored = torch.floor(tensor)
-
Ceiling elements to nearest integer:
ceiled = torch.ceil(tensor)
This comprehensive cheat sheet covers a wide range of tensor operations in PyTorch. Experiment with these operations to enhance your understanding and proficiency in working with tensors. For more advanced operations and details, refer to the official PyTorch documentation.
Feel free to use this cheat sheet as a reference guide for tensor operations in PyTorch!