TypeScript Cheat Sheet: Essential Code Examples
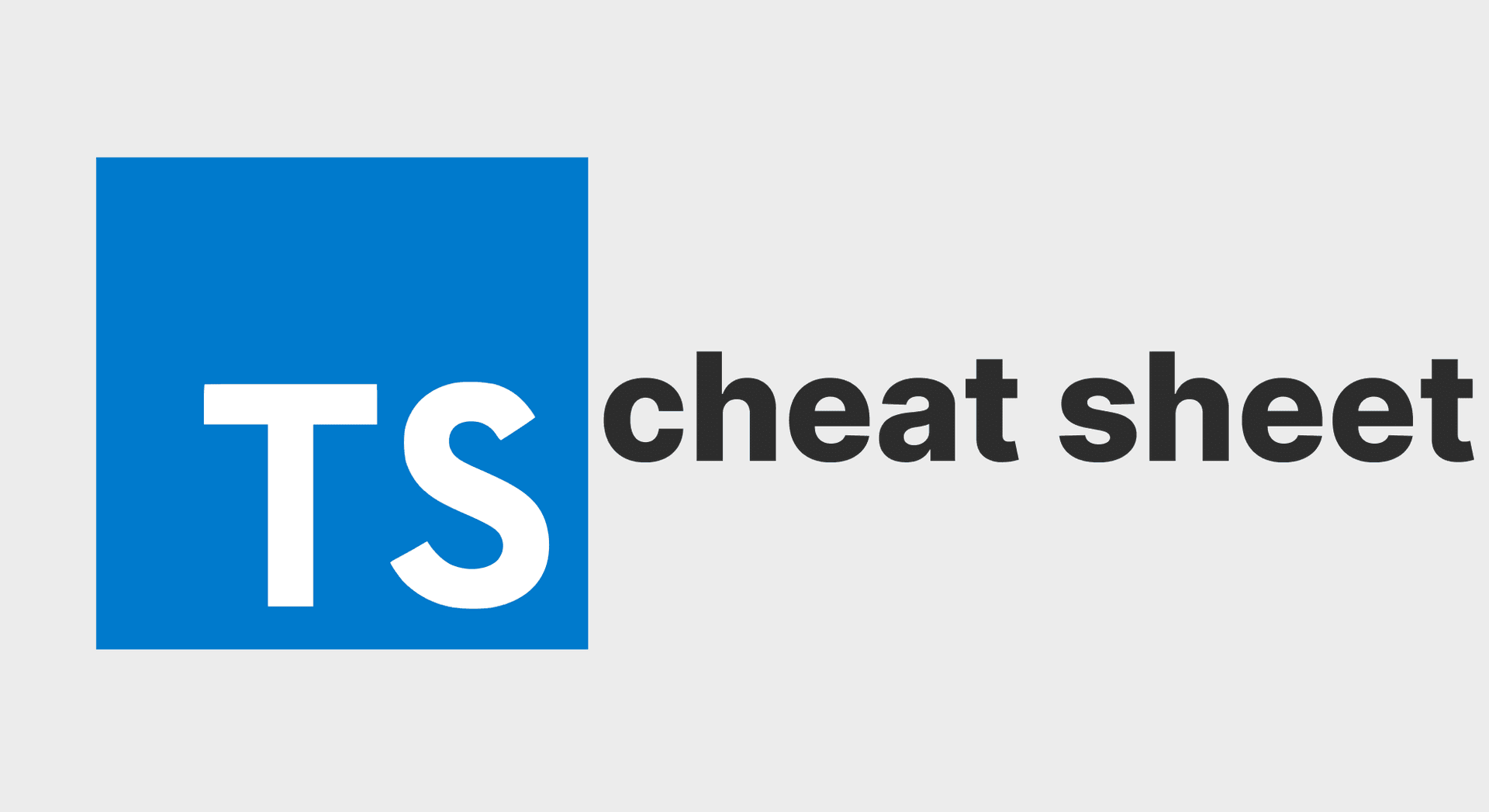
TypeScript Cheat Sheet: Essential Code Examples
TypeScript is a powerful superset of JavaScript that brings static typing and advanced features to your code. This cheat sheet provides a compilation of essential code snippets for common TypeScript concepts, making it a valuable reference for developers looking to enhance their coding skills.
Basic Types
Number
let age: number = 30;
String
let message: string = 'Hello, TypeScript!';
Boolean
let isActive: boolean = true;
Type Annotations
Function Parameter
function greet(name: string): string {
return `Hello, ${name}!`;
}
Object Property
interface Person {
firstName: string;
lastName: string;
}
function getFullName(person: Person): string {
return `${person.firstName} ${person.lastName}`;
}
Interfaces and Classes
Interface
interface Shape {
area(): number;
}
class Circle implements Shape {
constructor(private radius: number) {}
area(): number {
return Math.PI * this.radius * this.radius;
}
}
Class Inheritance
class Rectangle extends Shape {
constructor(private width: number, private height: number) {
super();
}
area(): number {
return this.width * this.height;
}
}
Enums
enum Color {
Red,
Green,
Blue
}
let selectedColor: Color = Color.Green;
Generics
function toArray<T>(arg: T): T[] {
return [arg];
}
let stringArray: string[] = toArray('Hello');
Type Assertion
let value: any = 'TypeScript';
let length: number = (value as string).length;
Union and Intersection Types
Union Type
let value: string | number;
value = 'Hello';
value = 42;
Intersection Type
interface Employee {
id: number;
name: string;
}
interface Department {
departmentId: number;
departmentName: string;
}
let employee: Employee & Department;
Enums and String Enums
Numeric Enum
enum Direction {
Up,
Down,
Left,
Right
}
String Enum
enum HttpStatus {
Ok = '200 OK',
NotFound = '404 Not Found',
InternalServerError = '500 Internal Server Error'
}
Modules and Namespace
Export and Import
// math.ts
export function add(a: number, b: number): number {
return a + b;
}
// app.ts
import { add } from './math';
Namespace
namespace Geometry {
export function area(radius: number): number {
return Math.PI * radius * radius;
}
}
Type Declarations
Type Definition
declare type Point = { x: number; y: number };
Ambient Declaration
declare module 'library' {
export function greet(name: string): void;
}
Conclusion
This TypeScript cheat sheet covers a wide range of essential code snippets for common programming concepts. Whether you're dealing with basic types, type annotations, interfaces, classes, enums, generics, or module system, these snippets will serve as valuable references to help you master TypeScript and build robust applications with confidence.